Set Up Encryption on Amazon SQS Delivery
To set up Encryption on Delivery to Amazon SQS, you must have access to Nayax Core and
Lynx API with the appropriate user permissions, Specifically the following roles:
- Lynx - SQS Encryption
To enable the encryption on the messages sent Amazon SQS you should mark the Enable Encryption
check box in the relevant configuration section as described in the previous sections.
Access the Lynx API
-
Enter your Nayax Core credentials.
Generate Encryption Keys
You will need the Actor ID (Operator ID)to generate encryption keys, which can be found in Nayax Core under Administration > Operator > Details tab.
- Click the Generate New Token button in the top-right corner of the Lynx API interface.
- Select the PUT /v1/actors/GenerateEncKey row to reveal more details
- Enter in
actorID
parameter value. - Click the Try it out! button to generate a new encryption key.
Encryption Key Usage and Refresh Guidelines
- If the Enable Encryption checkbox is marked in any SQS message delivery configuration within Nayax Core, all messages sent to the queue will be encrypted using the generated encryption key.
- The encryption key can be refreshed by repeating the steps above. Each new key will have an incremented
enc_ver
value, indicating the key version number.
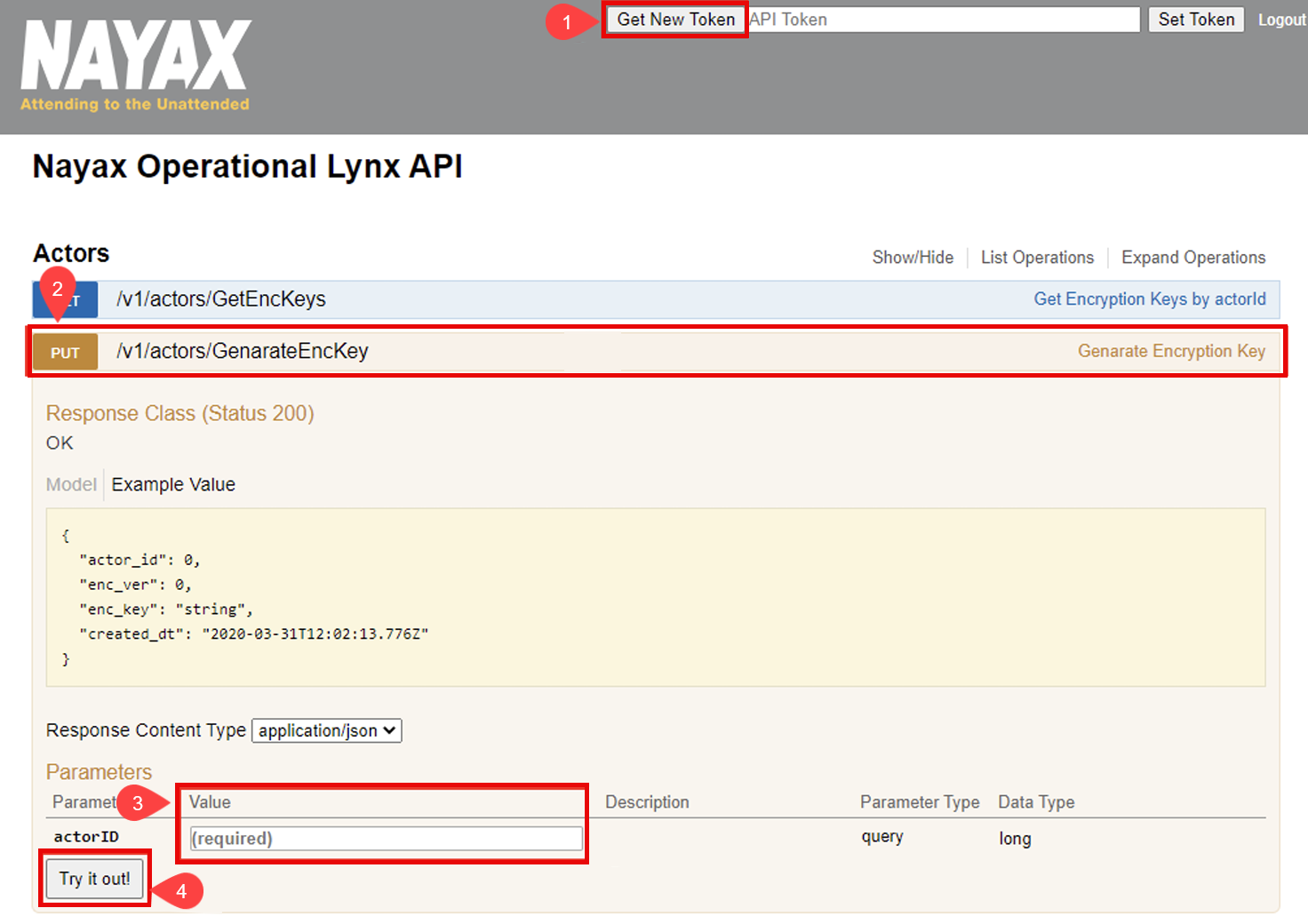
The Response will contain the following values:
Field Name | Description |
---|---|
actor_id | Auto generate unique id, represents the hierarchical entity in the hierarchy tree |
enc_ver | Numerator identifying Encryption Key Version |
enc_key | Encryption Key, Alphanumeric GUID used to encrypt Messages delivered to Amazon SQS |
created_dt | Encryption Key Creation Date and Time |
Example Response
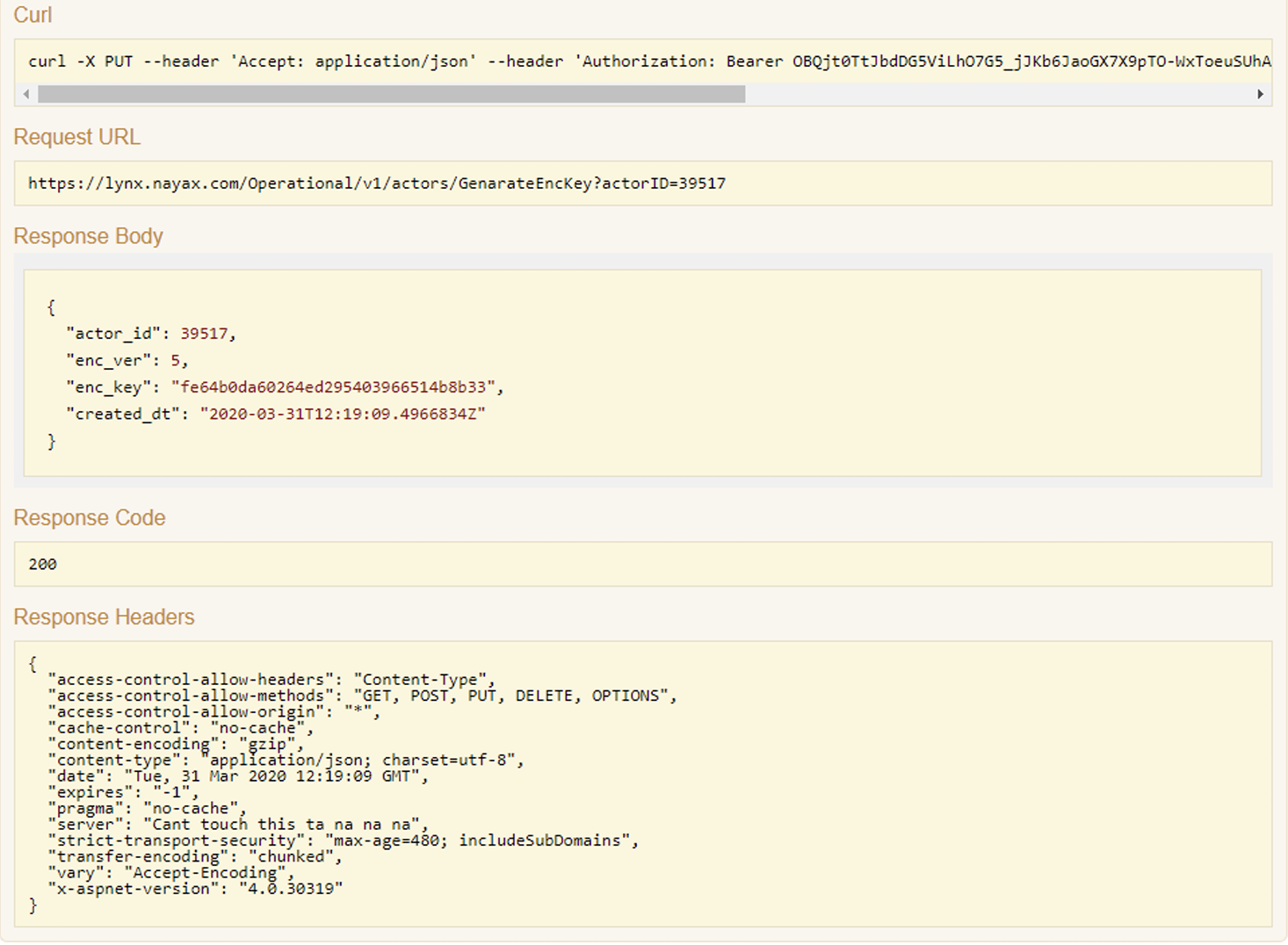
List Encryption Keys
You can retrieve all previously generated keys using the Lynx API's GET Encryption Keys option:
-
Click on GET /v1/actors/GetEncKeys row to reveal more details
-
Enter the
actorID
parameter value. -
Click the Try it out! button to view the list of generated keys.
By following these steps, you can ensure secure and encrypted message delivery to Amazon SQS.
Example Response:
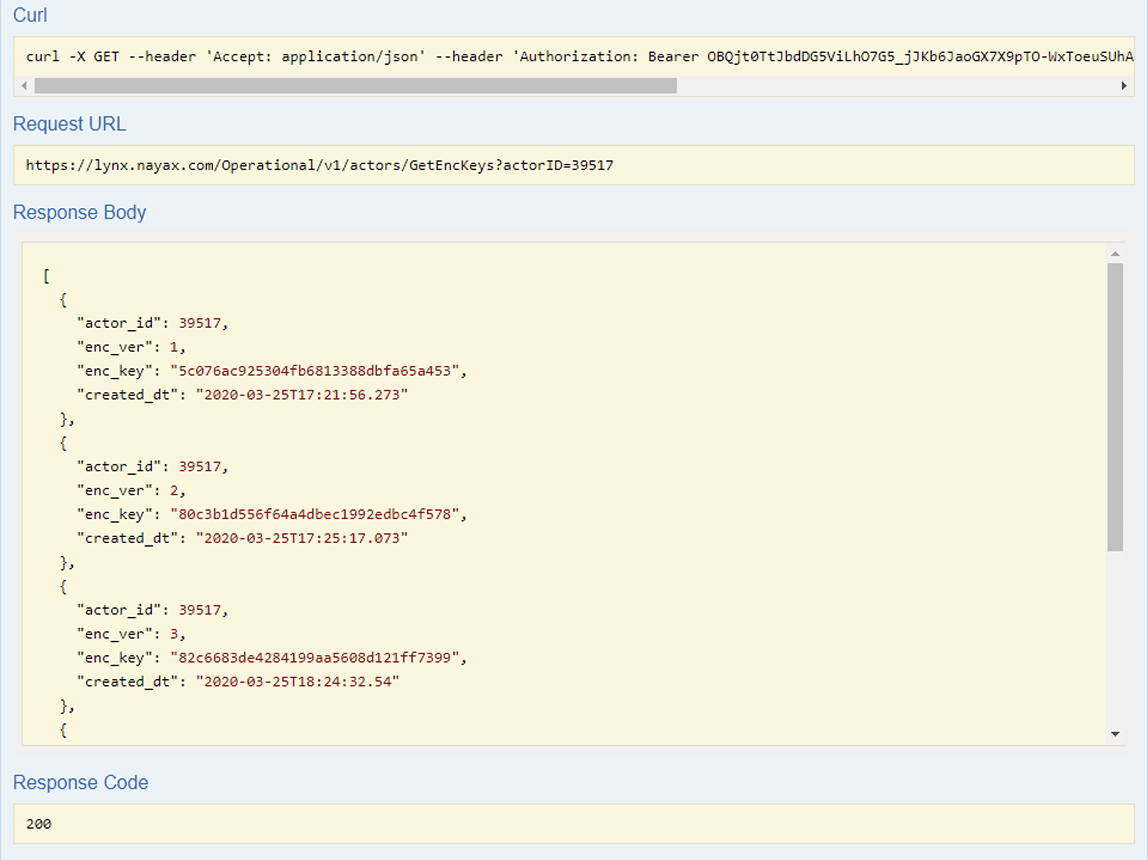
Decrypt Messages
You can also use the encryption key to perform the decryption of messages. See the code block below:
public string Decrypt(string cipherText)
{
using (Aes aesAlg = Aes.Create())
{
aesAlg.Key = Key;
aesAlg.IV = IV;
ICryptoTransform decryptor = aesAlg.CreateDecryptor(aesAlg.Key, aesAlg.IV);
using (MemoryStream msDecrypt = new MemoryStream(Convert.FromBase64String(cipherText)))
{
using (CryptoStream csDecrypt = new CryptoStream(msDecrypt, decryptor, CryptoStreamMode.Read))
{
using (StreamReader srDecrypt = new StreamReader(csDecrypt))
{
return srDecrypt.ReadToEnd();
}
}
}
}
}
}
// AES Encryption Example
string key = "0123456789abcdef"; // 16 bytes key
string iv = "abcdef9876543210"; // 16 bytes IV
AesEncryption aes = new AesEncryption(key, iv);
string original = "Hello, World!";
string decrypted = aes.Decrypt(encryptedMessage);
Where:
- It initializes the AES algorithm with a
key
and an initialization vector (IV
) and then creates adecryptor
. - The encrypted message, provided as a
Base64
string is converted back into bytes and read using aMemoryStream
andCryptoStream
with thedecryptor
. - The decrypted data is then read and returned as the original plaintext string.
The method essentially reverses the encryption process to retrieve the original message.
Updated about 1 month ago